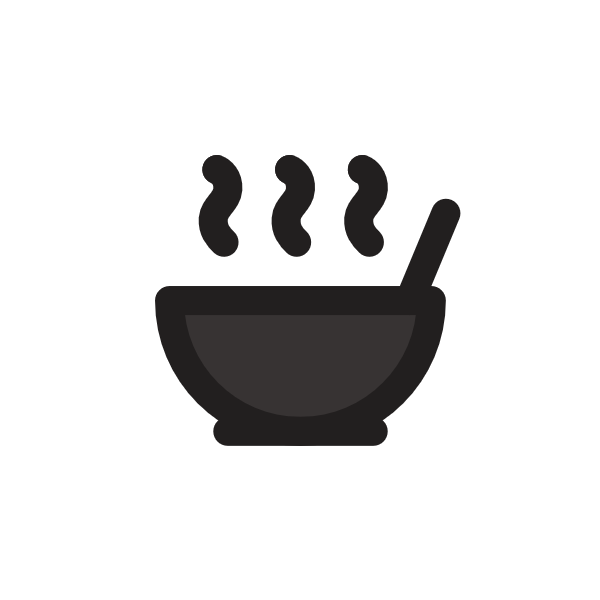
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Buffer overflow
Read the original article here.
The Forbidden Code: Unveiling Buffer Overflow Vulnerabilities
Welcome, aspiring digital artisans, to a deep dive into the underbelly of software security. In the shadows of standard programming courses lie techniques they often gloss over, deemed too complex, too dangerous, or simply not part of the curriculum. Today, we pull back the curtain on one of the most fundamental and historically significant vulnerabilities: the Buffer Overflow.
Understanding buffer overflows isn't just about finding flaws; it's about understanding the raw, physical layout of memory, the subtle dance between data and code, and the power that comes from manipulating that dance. It's a key skill for anyone seeking to truly master software security, whether you're building impenetrable defenses or exploring the weaknesses of existing systems.
What is a Buffer Overflow? The Fundamental Flaw
At its core, a buffer overflow (or buffer overrun) is a memory safety error. It occurs when a program tries to write more data into a fixed-size memory buffer than it was designed to hold. This excess data doesn't just disappear; it spills over into adjacent memory locations, overwriting whatever was stored there.
Buffer: A contiguous area of memory allocated to temporarily hold data while it is being moved or processed. Think of it like a bucket of a specific size.
Buffer Overflow (or Buffer Overrun): An anomaly where a program writes data beyond the allocated memory boundary of a buffer, overwriting adjacent memory locations.
Imagine you have a small cup (a buffer) designed to hold 8 ounces of liquid. If you try to pour 10 ounces into it, the extra 2 ounces will spill out, potentially affecting whatever is around the cup (adjacent memory).
This phenomenon often happens due to insufficient bounds checking – the act of verifying that the data being written fits within the buffer's allocated size. If the programmer assumes the input data will always be smaller than the buffer, a larger-than-expected input (often crafted maliciously) can trigger the overflow.
The consequences of overwriting adjacent memory can range from benign (like a program crash or incorrect results) to catastrophic (like gaining control over the program's execution or the entire system).
The Technical Mechanism: Memory Corruption
To understand buffer overflows technically, you need a basic grasp of how programs store data in memory. Memory is typically organized linearly, with different types of data stored in specific regions (like the stack, heap, and data segments). Buffers are allocated within these regions.
When data is written to a buffer, the program expects it to fit within the buffer's designated start and end addresses. If the write operation continues past the end address, it overwrites the bytes immediately following the buffer in memory.
Consider this simplified conceptual memory layout:
[ ... some data ... ]
[ Buffer A (8 bytes) ] <--- Overflow happens here
[ Variable B (2 bytes) ] <--- Overwritten data
[ ... other memory ... ]
If data written to "Buffer A" exceeds 8 bytes, the excess will overwrite the data belonging to "Variable B". What was originally stored in B is now replaced by bytes from the overflowed data.
The Root Cause: Lack of Bounds Checking
The programming languages most notoriously associated with buffer overflows are C and C++. Why? Because they are low-level languages that provide direct memory access and, critically, do not automatically perform bounds checking when dealing with arrays (which serve as built-in buffers) or pointers.
In C/C++, if you use a function like strcpy
to copy data into a buffer, the function will blindly copy bytes from the source string until it encounters a null terminator (\0
), regardless of the destination buffer's size. This is incredibly efficient but equally dangerous if the source string is larger than the destination buffer.
While C++'s Standard Template Library (STL) offers containers like std::vector
and std::string
with bounds-checked accessors (like .at()
), the core language and many common operations still allow unchecked memory access for performance reasons or backward compatibility with C. Using operator[]
on a std::vector
in C++, for example, does not perform bounds checking by default.
This lack of automatic protection makes C/C++ powerful for performance-critical tasks but also a breeding ground for memory safety issues like buffer overflows if the programmer isn't meticulously careful about managing buffer sizes.
Anatomy of an Overflow: A C Example
Let's break down the classic C example often used to illustrate a simple buffer overflow:
#include <string.h>
int main() {
char buffer_A[8]; // An 8-byte buffer
int variable_B = 1979; // A 2-byte integer (big-endian assumption from source)
// Assume buffer_A and variable_B are allocated adjacently in memory
printf("Initial value of B: %d\n", variable_B);
// **Vulnerable Operation:** strcpy does NOT check the size of buffer_A
strcpy(buffer_A, "excessive"); // "excessive" is 9 chars + null = 10 bytes
printf("Value of B after overflow: %d\n", variable_B);
return 0;
}
Initial State (Conceptual Memory):
Memory Address -> ... | [buffer_A bytes] | [variable_B bytes] | ...
Content -> ... | 00 00 00 00 00 00 00 00 | 07 BB | ...
(empty) (1979 in big-endian)
The Overflow:
The strcpy
function starts copying "excessive\0" (10 bytes) into buffer_A
(8 bytes).
strcpy(buffer_A, "excessive");
Memory Address -> ... | [buffer_A bytes] | [variable_B bytes] | ...
Content -> ... | 65 78 63 65 73 73 69 76 | 65 00 | ...
('e''x''c''e''s''s''i''v') ('e''\0') <--- Overflow spills into B
The last two bytes of the string ("e\0") overflow past the end of buffer_A
and overwrite the first two bytes of variable_B
.
Final State (Conceptual Memory):
Memory Address -> ... | [buffer_A bytes] | [variable_B bytes] | ...
Content -> ... | 65 78 63 65 73 73 69 76 | 65 00 | ...
('e''x''c''e''s''s''i''v') ('e''\0')
The original value of variable_B
(1979, or 0x07BB
) has been replaced by the bytes 0x6500
(the ASCII value of 'e' followed by the null terminator). Assuming a system where int
is read from these bytes, 0x6500
corresponds to the decimal number 25856.
This is a simple example showing data corruption. While sometimes this just crashes the program (e.g., a segmentation fault if the overflow writes to protected memory), skilled attackers can strategically overwrite specific data to achieve more malicious goals.
Safer Alternatives (In C/C++):
To prevent this specific overflow, the programmer should use functions that are aware of the destination buffer's size.
strncpy
: Copies up ton
characters. However, it doesn't guarantee null-termination if the source string is longer than or equal ton
. This means the resulting buffer might not be a valid C-style string, which can cause issues later.strlcpy
: A safer alternative (available on systems like OpenBSD and often provided in porting libraries). It takes the full size of the destination buffer, guarantees null-termination if the source fits, and copies at mostsize - 1
bytes. This prevents the overflow.
// Using strlcpy (if available)
// strlcpy(buffer_A, "excessive", sizeof(buffer_A));
// Using strncpy (with care to null-terminate)
strncpy(buffer_A, "excessive", sizeof(buffer_A) - 1); // Copy at most 7 bytes
buffer_A[sizeof(buffer_A) - 1] = '\0'; // Manually null-terminate
// Or, ideally, use safer string handling functions from libraries
// or C++ string objects.
The key takeaway: Always be size-aware when writing data into fixed-size buffers.
Stepping into the Underground: Exploitation
Understanding how an overflow happens is the first step. The "forbidden code" aspect comes from understanding how these memory corruption vulnerabilities can be exploited to gain control over a program, leading to unauthorized access, privilege escalation, or arbitrary code execution.
Exploitation techniques are highly dependent on:
- The Vulnerable Code Location: Is the buffer on the stack (used for local variables, function calls) or the heap (used for dynamic memory allocation)?
- The Target Architecture & OS: Memory layout, calling conventions, and security features vary.
- The Specific Program State: What data structures or pointers are located adjacent to the buffer?
Stack Overflows: Hijacking Control Flow
The stack is a fundamental memory region managed during function calls. When a function is called, a stack frame is created for it. This frame typically contains:
- Function arguments
- Local variables (including buffers)
- Saved register values
- The return address: the memory address the program should jump back to after the function finishes.
Stack Frame: A data structure that stores information about a single execution of a subroutine (function). It is pushed onto the call stack when the subroutine is called and popped off when the subroutine returns.
A stack-based buffer overflow occurs when a buffer allocated on the stack is overflowed. The critical point here is that local variables and the return address are often located close to each other within the same stack frame or adjacent frames.
Common Stack Exploitation Goals via Overwriting:
- Overwrite Local Variables: Simply changing the value of a variable near the buffer to alter program logic (e.g., changing a boolean flag, an ID, a counter).
- Overwrite the Return Address: This is the most common technique for arbitrary code execution. By overflowing a buffer located below the return address on the stack, an attacker can overwrite the return address pointer with an address of their choosing. When the vulnerable function returns, instead of going back to the legitimate caller, the program jumps to the address supplied by the attacker.
- Overwrite Function Pointers or Exception Handlers: If the program uses function pointers (variables holding memory addresses of functions) or has registered exception handlers on the stack, overflowing a buffer nearby can overwrite these pointers to point to attacker-controlled code.
- Overwrite Variables in Other Stack Frames: Less common, but possible if the buffer is located such that it can overflow into the stack frame of a calling function and corrupt its variables or pointers.
The Prize: Shellcode
When an attacker successfully overwrites a control flow pointer (like the return address or a function pointer), they typically want execution to jump to their own code. This malicious code is often referred to as shellcode.
Shellcode: A small piece of assembly code, often written to launch a command shell on the target system, but can perform any arbitrary task chosen by the attacker (e.g., download and execute malware, steal data, add a user account). It's loaded into memory by the attacker's input and executed as a result of the exploitation.
The attacker injects the shellcode into the vulnerable program's memory (often within the buffer itself or immediately following it in the malicious input). The overwritten pointer is then set to the memory address where the shellcode resides.
Heap Overflows: Corrupting Data Structures
The heap is a region of memory used for dynamic memory allocation (using functions like malloc
, calloc
, new
). Unlike the stack, its structure is less rigid and depends heavily on the memory manager used by the operating system and C runtime library. The heap typically holds program data structures, objects, and dynamically created buffers.
A heap overflow occurs when a buffer allocated on the heap is overflowed. Since the heap doesn't have a standard "return address" like the stack, heap exploitation focuses on corrupting data structures used by the memory manager itself or other program data structures stored on the heap.
Canonical Heap Exploitation Technique:
A classic technique involves overflowing a heap buffer to overwrite the metadata used by the malloc
library to manage allocated chunks of memory. This metadata often includes pointers used to link free chunks together. By corrupting these pointers, an attacker can trick malloc
or free
into writing an arbitrary value to an arbitrary memory location during a subsequent heap operation.
This arbitrary write primitive can then be used to overwrite a function pointer within the program's data section (not on the stack or heap, but in a different memory region). Overwriting a function pointer allows the attacker to divert program execution when that function is called.
Heap exploitation can be more complex and memory-manager-specific than stack exploitation but is equally dangerous and can be used to bypass stack-based defenses.
Obstacles and Evasion: Barriers to Exploitation
Modern systems and careful programmers employ countermeasures to make exploitation harder. Attackers, in turn, develop techniques to bypass these defenses.
Initial Barriers (Often Input Filtering):
Vulnerable applications might attempt to filter or manipulate user input before it's copied into a buffer. This could include:
- Converting input to upper or lower case.
- Removing "metacharacters" (like quotes, backslashes, command separators).
- Filtering out null bytes (
\0
), which are crucial for C-string termination and often appear in memory addresses. - Allowing only alphanumeric characters.
Attacker Bypass Techniques:
Skilled attackers have developed ways to craft shellcode and exploit payloads that function despite these manipulations:
- Alphanumeric Shellcode: Crafting shellcode using only alphanumeric characters and a few allowed symbols. This is complex but possible using self-modifying code techniques.
- Polymorphic Code: Shellcode that changes its appearance (the bytes themselves) with each execution while retaining the same functionality. This helps evade signature-based intrusion detection systems.
- Self-Modifying Code: Shellcode that modifies parts of itself in memory during execution. This can be used to decode restricted bytes or perform complex operations not possible with a static payload.
- Return-to-libc (or Return-Oriented Programming - ROP): Instead of injecting shellcode, the attacker overwrites control flow pointers (like the return address) to jump to small sequences of existing, legitimate instructions already present in the program's memory (often within standard libraries like
libc
). These sequences (called "gadgets") typically end with aret
instruction, which pops the next address off the stack and jumps to it. By chaining together multiple gadgets, the attacker can perform arbitrary actions without injecting any new code. This technique is particularly effective against defenses that prevent code execution on the stack or heap.
Understanding these bypass techniques is essential for building robust defenses and analyzing real-world attacks.
Advanced Exploitation Techniques: Getting Reliable Execution
Even without input filtering, exploitation faces practical challenges:
- Memory Addresses: Where exactly is the buffer or the target return address located in memory? This can vary slightly between systems or even between program runs.
- Null Bytes: Memory addresses (especially on 32-bit systems where they might start with
0x00
) often contain null bytes (\0
). If the vulnerable copy function (likestrcpy
) stops copying at the first null byte, the attacker's payload might be truncated.
Here are two classic techniques used to overcome these challenges:
The Classic: The NOP Sled
The NOP sled is one of the oldest techniques for stack buffer overflows, designed to deal with the uncertainty of the exact shellcode location.
NOP (No-Operation): A machine instruction that does nothing except advance the instruction pointer to the next instruction. Conceptually, it's like a "do nothing" step in the program's execution.
Mechanism:
The attacker crafts a large input consisting of:
- A long sequence of NOP instructions (the "NOP sled").
- The actual shellcode.
- An overwritten return address pointing anywhere within the NOP sled.
When the vulnerable function returns, execution jumps to the address in the overwritten return address.
If this address is within the NOP sled, the processor simply executes NOP after NOP, "sliding" down the sled until it hits the shellcode.
Execution then continues into the shellcode.
Conceptual Stack with NOP Sled:
[ ... legitimate data ... ]
[ Vulnerable Buffer + Overflowed Region ] -> [ NOP NOP NOP NOP ... (NOP Sled) ] [ Shellcode ] [ Overwritten Return Address -> points into NOP sled ]
[ ... stack frame of caller ... ]
Advantages: The attacker doesn't need to guess the exact address of the shellcode, only an address somewhere within the large NOP sled region.
Disadvantages:
- Requires a significant amount of memory for the NOP sled, which might not always be available in the target buffer or adjacent stack space.
- NOP sleds are easily detectable by intrusion detection systems or stack protection mechanisms looking for long sequences of NOPs. Attackers sometimes use sequences of other benign instructions instead of just NOPs to evade this detection.
Reliability via Jump-to-Register
This technique offers greater reliability and doesn't require guessing stack offsets or large NOP sleds.
Mechanism:
- The attacker injects the shellcode into a predictable location, often within the vulnerable buffer itself.
- The attacker then finds a specific instruction sequence already present in the program's memory (a "gadget"), usually in loaded libraries. A common example on x86 is the
jmp esp
instruction (jump to the address currently stored in the ESP register, which typically points to the top of the stack, where the attacker's data/shellcode is). - The attacker overflows the buffer to overwrite the return address with the memory address of this
jmp esp
(or similar) instruction.
Conceptual Stack with Jump-to-Register:
[ ... legitimate data ... ]
[ Vulnerable Buffer ] -> [ Shellcode ]
[ Overwritten Return Address -> points to address of 'jmp esp' gadget ]
[ ... stack frame of caller ... ]
Somewhere else in memory (e.g., a library):
[ Address 0xDEADBEEF ] -> [ jmp esp instruction ]
- When the vulnerable function returns, execution jumps to the
jmp esp
gadget. - The
jmp esp
instruction then redirects execution to the top of the stack, which is now controlled by the attacker and contains their shellcode.
Advantages: Highly reliable as it relies on the program jumping to a known, static address (jmp esp
gadget) which then jumps to the shellcode whose relative position on the stack is known to the attacker. Doesn't need NOPs.
Handling Null Bytes with DLL Trampolining:
On 32-bit Windows, executable code often loads below address 0x01000000
, meaning addresses often start with 0x00
, which is a null byte. Overwriting a return address with an address containing 0x00
can truncate the copied payload if the copy function is null-byte sensitive. However, Dynamic Link Libraries (DLLs) on Windows are typically loaded at higher memory addresses (above 0x01000000
), and their addresses usually do not contain null bytes.
Attackers can find jmp esp
(or similar) gadgets within these DLLs. By overwriting the return address with the address of a gadget in a DLL, they avoid the null byte issue, ensuring the full payload (including the shellcode placed after the overwritten return address) is written to the stack before the jump occurs. This specific technique is sometimes called "DLL Trampolining".
This reliability makes the jump-to-register technique, often involving ROP variants, the preferred method for modern buffer overflow exploits, especially in worms or automated attacks.
Fortifying the Gates: Protective Countermeasures
The prevalence and severity of buffer overflows have driven the development of various countermeasures, both in software development practices and operating system features. Understanding these defenses is crucial for building secure software and for understanding why older exploitation techniques might fail today.
Choice of Programming Language
The most fundamental defense is to use programming languages that are inherently less susceptible to buffer overflows due to built-in memory safety features.
- Safe Languages: Languages like Python, Java, C#, Rust, Ada, Eiffel, Lisp, and most interpreted languages perform automatic bounds checking on array and string accesses at runtime (and sometimes compile-time). Attempts to write beyond a buffer's boundary result in a well-defined error condition (like an exception) rather than silent memory corruption. Rust, in particular, achieves memory safety and thread safety without a garbage collector, providing performance competitive with C/C++ while preventing common vulnerabilities like buffer overflows and data races through strict compile-time checks.
- Managed Environments: Languages running within managed environments like the Java Virtual Machine (JVM) or the .NET Framework benefit from the runtime's enforcement of memory safety rules.
While switching languages isn't always feasible for legacy code, choosing safer languages for new development significantly reduces the risk of introducing these vulnerabilities.
Use of Safe Libraries
For languages like C and C++ where raw memory access is common, relying on carefully designed "safe" libraries for common tasks like string manipulation and buffer handling is essential.
Avoiding notorious unsafe functions like gets
, strcpy
, strcat
, sprintf
, and scanf
(which do not take buffer size limits) is a baseline requirement for secure C/C++ coding. Replace them with size-aware alternatives:
fgets
(for input)strncpy
(use carefully, remember null termination)strncat
snprintf
strlcpy
,strlcat
(if available or ported)
Using C++ standard library classes like std::string
and std::vector
is generally safer than C-style arrays and character pointers, provided safe access methods (.at()
) are used where bounds checking is desired, or careful size management is maintained.
Dedicated "safe string" libraries have also been developed, although widespread adoption can be challenging.
Runtime Protections: Detecting and Mitigating Attacks
Modern operating systems and compilers include features designed to detect or prevent buffer overflow exploits at runtime.
Stack Protection (Stack Canaries)
This technique focuses on detecting whether the stack has been corrupted before a function returns.
Stack Canary: A known value (often random) placed on the stack between a buffer and control-sensitive data (like the return address). Before a function returns, the program checks if the canary value has been altered. If it has, it indicates a potential buffer overflow, and the program is typically terminated to prevent exploitation.
The canary acts like a tripwire. If an attacker tries to overflow a buffer to reach the return address, they will likely overwrite the canary value on the way. When the function epilogue checks the canary, it finds the corrupted value and aborts the program.
Implementations include:
- StackGuard/ProPolice (GCC): Compiler features that add canaries automatically.
- Microsoft's /GS compiler flag: Adds security checks, including stack canaries.
While effective against many stack overflows, stack canaries aren't a perfect solution. Attackers might find ways to leak the canary value or exploit vulnerabilities that don't require overwriting the canary.
Pointer Protection
This defense aims to make it harder for attackers to reliably overwrite and use pointers.
Mechanism: Pointers are encoded (e.g., XORed with a random value) before being stored in memory. Before a pointer is used, it is decoded. If an attacker overwrites an encoded pointer without knowing the encoding key, the decoded pointer will likely be an invalid or unpredictable address, causing the program to crash rather than jump to the attacker's desired location.
Microsoft's SafeSEH and pointer encoding features are examples. Challenges include managing the encoding key securely and protecting against partial pointer overwrites (e.g., if only the lower bytes of an address are overwritten due to a null byte).
Executable Space Protection (DEP/NX)
This is a fundamental defense that prevents code from being executed in memory regions designated for data (like the stack and the heap).
Data Execution Prevention (DEP) / No eXecute (NX bit): A system security feature that marks memory pages as either executable OR writable, but not both simultaneously (W^X: Write XOR Execute). This prevents attackers from injecting shellcode into data areas (like the stack or heap) and then executing it.
Hardware support (the NX or XD bit on modern CPUs) combined with OS enforcement allows the memory manager to set permissions on pages. If a program tries to execute instructions from a page marked as non-executable (e.g., a stack page containing attacker-injected shellcode), the processor generates an exception, and the OS terminates the program.
DEP/NX is a powerful defense against traditional shellcode injection attacks. However, it does not prevent return-oriented programming (ROP) attacks, as ROP executes existing, legitimate code sequences already present in executable memory segments (like the .text
section).
Address Space Layout Randomization (ASLR)
ASLR makes exploitation harder by randomizing the memory addresses where key parts of a program are loaded.
Address Space Layout Randomization (ASLR): A computer security technique that randomly arranges the positions of a process's address space, including the base of the executable, libraries, heap, and stack, at runtime.
With ASLR enabled, the addresses of functions, libraries (like libc
), and the stack change every time the program runs. This means an attacker cannot rely on hardcoded memory addresses for their exploits (e.g., the fixed address of a jmp esp
gadget or the location of a specific function in libc
for a return-to-libc attack).
Impact:
- Makes Exploit Development Harder: Attackers must find ways to leak memory addresses or bypass the randomization.
- Prevents Worms: Exploits relying on fixed addresses won't work reliably across different systems or even different runs on the same system.
ASLR is often combined with DEP/NX. While not insurmountable (information leakage vulnerabilities can sometimes defeat ASLR), it significantly raises the bar for attackers.
Capability Hardware Enhanced RISC Instructions (CHERI)
A more advanced, hardware-level approach still under development. CHERI modifies the processor architecture and instruction set to introduce "capabilities" – secure pointers that include bounds and permissions metadata enforced by the hardware. This aims to prevent spatial memory errors (like buffer overflows) and temporal errors (like use-after-free) at the hardware level.
Network-Level Detection (DPI)
While not a replacement for host-based defenses, some network intrusion detection/prevention systems (IDS/IPS) use Deep Packet Inspection (DPI) to look for signatures of known buffer overflow attacks (e.g., long sequences of NOPs, specific shellcode patterns) in network traffic.
However, this is a weak defense against sophisticated attackers who can use polymorphic or encrypted shellcode, or leverage techniques like ROP that don't involve injecting easily identifiable patterns.
Finding Vulnerabilities (Testing)
Identifying buffer overflow vulnerabilities before deployment is a critical defensive measure. Techniques include:
- Fuzzing: Feeding a program with large amounts of semi-random or malformed data to trigger unexpected behavior or crashes, which can indicate buffer overflows.
- Static Analysis: Using specialized tools to analyze source code without executing it, identifying potentially unsafe coding patterns (like using
strcpy
without bounds checking). - Dynamic Analysis: Analyzing the program while it runs, monitoring memory access for out-of-bounds writes.
- Manual Code Review: Experienced security auditors can often spot subtle vulnerabilities missed by automated tools.
Fixing these vulnerabilities during development is far more effective than trying to detect attacks in the wild.
A Brief History of a Persistent Problem
Buffer overflows aren't a new phenomenon. They've been known for decades and have played a pivotal role in the history of computer security:
- 1972: The concept was documented in a US Air Force report, describing how improper address checking could allow users to overwrite parts of the operating system ("monitor") to gain control.
- 1988: The Morris Worm, one of the first major internet worms, used a buffer overflow in the
fingerd
service (specifically via a vulnerablegets
call) as one of its propagation methods. This event brought buffer overflows to mainstream attention within the nascent internet community. - 1995-1996: Rediscovery and detailed public documentation emerged. Thomas Lopatic posted about them on the Bugtraq mailing list. Crucially, Elias Levy (Aleph One) published the seminal paper "Smashing the Stack for Fun and Profit" in Phrack magazine, providing a step-by-step guide to stack overflow exploitation, which became a foundational text for a generation of security researchers and attackers.
- Early 2000s: Buffer overflows were exploited by widespread internet worms like Code Red (2001, targeting Microsoft IIS web server) and SQL Slammer (2003, targeting Microsoft SQL Server).
- Gaming Consoles: Buffer overflows have been famously used to bypass security measures on gaming consoles, allowing users to run unofficial "homebrew" software (e.g., exploits involving The Legend of Zelda: Twilight Princess on the Wii, exploits for Xbox and PlayStation 2).
This history underscores that despite decades of countermeasures, buffer overflows remain a relevant threat, particularly in codebases written in vulnerable languages or in embedded systems where advanced defenses might not be fully deployed.
Conclusion: Mastering Memory for Defense and Discovery
Buffer overflows are not just security vulnerabilities; they are direct consequences of how software interacts with memory at a low level. Understanding them requires delving into the architecture of computing, the nuances of programming languages, and the clever ways attackers and defenders manipulate program execution.
In the context of "The Forbidden Code," learning about buffer overflows is paramount. It equips you with:
- The ability to write more secure code: By understanding why functions like
strcpy
are dangerous and habitually using safer alternatives or languages. - The knowledge to identify vulnerabilities: Both manually through code review and by understanding how tools like fuzzers work.
- The insight to analyze and understand attacks: Deconstructing how exploits work, from simple return address overwrites to complex ROP chains bypassing ASLR and DEP.
- The foundation for understanding other memory safety issues: Buffer overflows are just one type; others like heap overflows, integer overflows, format string bugs, and use-after-free vulnerabilities share similar underlying principles of memory corruption.
While the landscape of exploitation and defense is constantly evolving with new techniques and countermeasures, the fundamental principles of buffer overflows remain a cornerstone of cybersecurity knowledge. Mastering this "underground" technique is essential, not just for offensive purposes, but for building the truly robust and resilient systems of the future. Now that you understand the theory, the real learning comes from seeing them in action and, ideally, practicing exploiting them in controlled, safe environments.